Grid System
The Choose Energy custom grid system is intended to be a flexible backbone for all our designs, providing a consistent layout pattern across all our web properties and all screen sizes.
Our grid system is designed to be used to create a set of blocks or tiles of standardized sizes that can be mixed and matched in various patterns to create more complex layouts for content.
About Our Grid System
Our custom grid is built off the foundations of the Bourboun Neat grid system. We use Neat mixins to drive most of the logic for our grids and then created our own modified versions of some of the column mixins to meet our specifc need for fixed-width blocks.
Our custom grid mixins can be found in the _grid.scss
file in the mixins directory. The inline documentation cover details about implementation as well as the allowed parameters for each mixin. All the grid values that can be passed in as parameters are stored in the _variable.scss
file. Do not pass in values for the grid outside of these variables as they cannot be guaranteed to work with our breakpoints otherwise!
Additional information about the availble options for the Bourboun Neat grid can be found in their execelent documentation. In general, we haven't needed to use many of these extra mixins or functions, but some may come in handy for unusual layouts.
How to Use
Using the grid system mixins to create content modules is pretty straightforward. The parent container element should always have the grid-container
mixin applied - amongst other things this mixin clears floats, centers the element in the page frame, and most importantly, sets the appropriate container width for each breakpoint.
Grid Columns
The actual block elements should have the grid-columns()
mixin applied. This mixin takes a couple parameters that adjust how the block is displayed. The first parameter is required and determines the number of grid columns the block spans. Including the omega()
mixin on the last item on any row will remove the unneeded right margin and ensure the block stays on the correct line.
@include grid-container;
@include grid-columns( 2 );
@include grid-columns( 1 );
@include omega();
.ce-exampleModule {
@include grid-container;
}
.example-block-1 {
@include grid-columns( 2 );
}
.example-block-2 {
@include grid-columns( 1 );
@include omega();
}
Grid Heights
The second optinal parameter sets a fixed height for the block to force it square - use short
for a single column square block and tall
for a 2 column block. If no parameter is passed in for the height, the block will default to a flexible height that fits the content.
@include grid-columns( 2, tall );
@include grid-columns( 1, short );
.ce-exampleModule {
@include grid-container;
}
.example-block-1 {
@include grid-columns( 2 );
}
.example-block-2 {
@include grid-columns( 1 );
@include omega();
}
You can also use the flexbox utility mixins in conjunction with the grid mixins to align content within fixed height blocks or force side-by-side blocks to match in height.
This block has a lot of text. It's going to force all the other blocks in the row to match it's height.
Minimal text centered vertically in the block.
Headline
.ce-exampleModule {
@include grid-container;
@include omega(3n);
@include flexbox-same-height;
}
.example-block-1 {
@include grid-columns( 1 );
}
.example-block-2 {
@include grid-columns( 1 );
@include flexbox-center-vertically;
}
.example-block-3 {
@include grid-columns( 1 );
@include flexbox-center;
}
Grid Gutters
The gutters between each block in the grid are always 10px/1rem - no exceptions! These gutters are set automatically by the grid-column()
mixin and the values are stored in the _variables.scss
file.
Block Padding
Each block created using the grid mixins will default to a 30px/3rem padding on all sides. This should be maintained as much as possible to ensure content aligns between blocks.
Blocks with no background or border may alternatively remove the padding on the side margins to align the text with the edge of other blocks. This should only be done if there is a large enough block of text to make the alignment obvious.
There is a 1rem gutter between each block...
and 3rem of padding within each block.
This applies to bordered or solid colored blocks.
This block has no side padding
Since there is no border or background, we can override the default padding on the sides of the block to allow the text to align with the edge of the nearby block. This is an optional layout choice, but one that is recommended if there are large blocks of text.
.ce-exampleModule {
@include grid-container;
@include flexbox-same-height;
}
.example-block-1 {
@include grid-columns( 1 );
}
.example-block-2 {
@include grid-columns( 3 );
padding: $grid-padding 0;
}
Responsive Design
Our grid system creates a set of up to 4 columns of blocks or tiles on desktop layouts. For tablets or smaller laptop screens, that drops to a max of 2 columns. On mobile devices, all blocks span a single, full-width column.
When creating blocks within a module, use the @include media()
mixin from Bourboun Neat to create alternate layouts for differing screen sizes. All the breakpoint values are stored in variables in the _variable.scss
file and these are the only parameters that should be passed into the media mixin.
On a desktop device, these three blocks will all show up in a row.
On a tablet, these first two blocks will span 2 columns and apear stacked.
This block will not be displayed on tablet or mobile devices.
.ce-exampleModule {
@include grid-container;
}
.example-block-1 {
@include grid-columns( 1, short );
@include media( $tablet ) {
@include span-columns-fixed( 2, $column-tablet );
}
}
.example-block-3 {
@include media( $tablet-down ) {
display: none;
}
}
The grid system is not designed to allow nested grids, so the smallest size any grid item should be is a 1 column block. Within an individual block, any further grouping of content should be done on a per-module basis, although even this should be done sparingly to avoid clutter.
Grid Utilities
There are also a handful of grid utility mixins that provide shortcuts for creating common types of blocks. These include headline blocks, block with images, and blocks containing icons.
Headline block with an icon block next to it
A block with a background image
The background image is set to cover the whole block, regardless of size.
A block with a content image inside it.
The horizontal axis of the image is set to fill 100% of the block (and crop on the vertial axis).
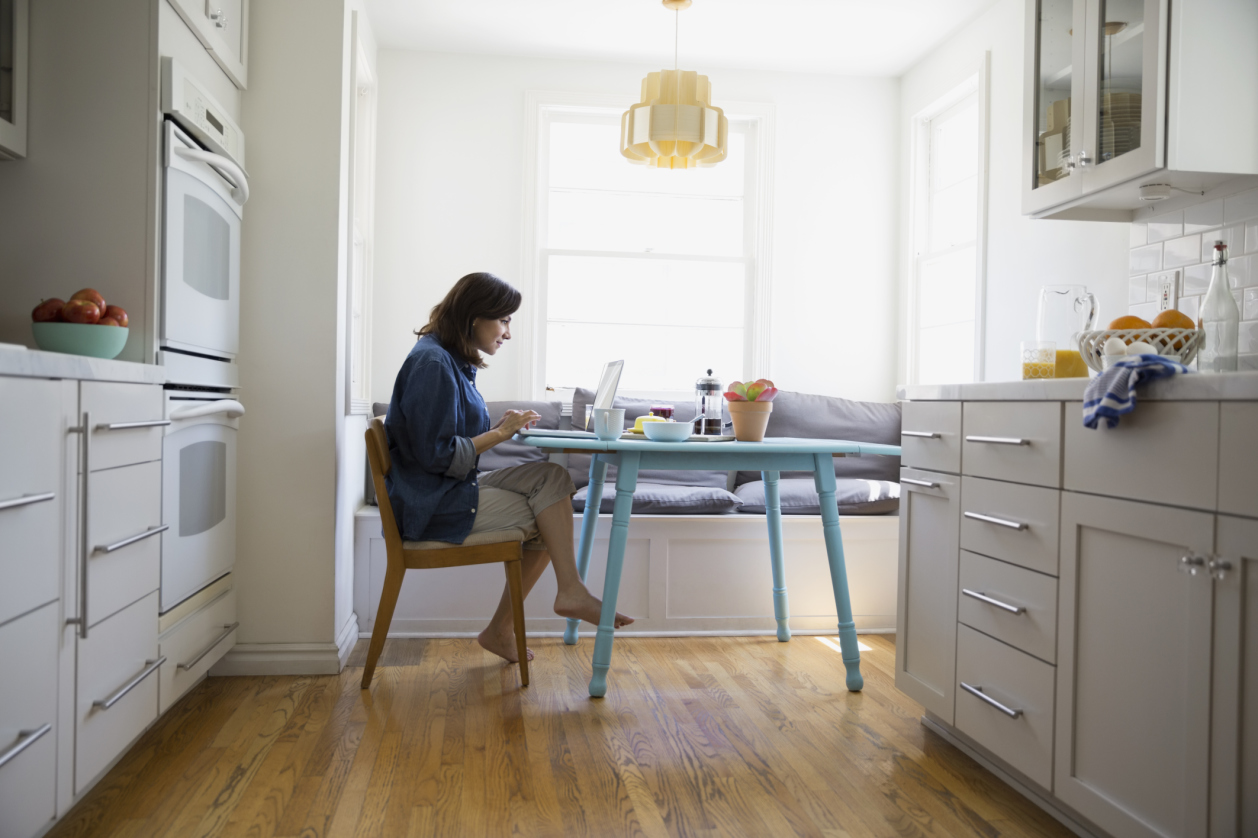
.ce-exampleModule {
@include grid-container;
}
.headline-block {
@include grid-columns( 2, short );
@include grid-headline;
}
.icon-block {
@include grid-columns( 1, short );
@include grid-bigIcon;
}
.background-image-block {
@include grid-columns( 2, short );
@include grid-bgImage;
}
.content-image-block {
@include grid-columns( 2, short );
@include grid-image( horizontal);
}